Craps Javascript Code
In this step-by-step tutorial we create a simple MDN Breakout game written entirely in pure JavaScript and rendered on HTML5 <canvas>
.
I'm developing a small craps game in C, and my C skills are a bit rusty. I really need someone to review my code to ensure that I have correctly implemented the game according to the rules. Game rules: The player or shooter rolls a pair of standard dice If the sum is 7 or 11 the game is won; If the sum is 2, 3 or 12 the game is lost. I deleted it out on accident when I put in my name header for class. I can't believe it was something that simple!! Thanks you guys! Consider the game of craps. Suppose that rolling a “7 or 11” is a success but everything else is a failure. What is the probability that your first failure is after 10 rolls? I'm not sure whether this is a binomial or geometric distribution. How should I approach this problem? (Using R by the way, so pbinom, pgeom, etc.). Craps is a fairly simple dice game often played in casinos. Even if you aren't a gambler (which I'm not), it's still a fairly interesting game. Here's the rules: At the start of a game of Craps there's what is called the come-out round. The player rolls two d6s (six-sided die) and the two die rolls are added.
Every step has editable, live samples available to play with so you can see what the intermediate stages should look like. You will learn the basics of using the <canvas>
element to implement fundamental game mechanics like rendering and moving images, collision detection, control mechanisms, and winning and losing states.
To get the most out of this series of articles you should already have basic to intermediate JavaScript knowledge. After working through this tutorial you should be able to build your own simple Web games.
Lesson details
All the lessons — and the different versions of the MDN Breakout game we are building together — are available on GitHub:
Starting with pure JavaScript is the best way to get a solid knowledge of web game development. After that, you can pick any framework you like and use it for your projects. Frameworks are just tools built with the JavaScript language; so even if you plan on working with them, it's good to learn about the language itself first to know what exactly is going on under the hood. Frameworks speed up development time and help take care of boring parts of the game, but if something is not working as expected, you can always try to debug that or just write your own solutions in pure JavaScript.
Note: If you are interested in learning about 2D web game development using a game library, consult this series' counterpart, 2D breakout game using Phaser.
Note: This series of articles can be used as material for hands-on game development workshops. You can also make use of the Gamedev Canvas Content Kit based on this tutorial if you want to give a talk about game development in general.
Next steps
Ok, let's get started! Head to the first chapter— Create the Canvas and draw on it.
Loops can execute a block of code as long as a specified condition is true.
The While Loop
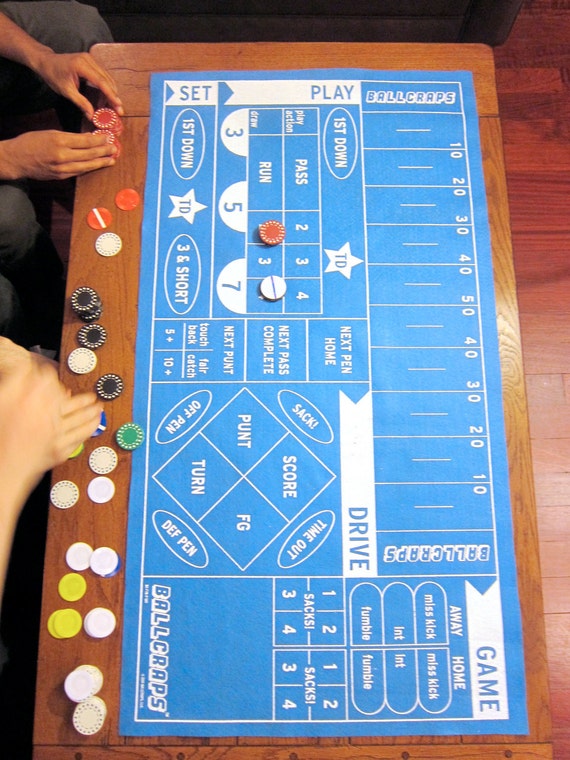
The while
loop loops through a block of code as long as a specified condition is true.
Syntax
// code block to be executed
}
Example
In the following example, the code in the loop will run, over and over again, as long as a variable (i) is less than 10:
Example
text += 'The number is ' + i;
i++;
}

If you forget to increase the variable used in the condition, the loop will never end. This will crash your browser.
The Do/While Loop
The do/while
loop is a variant of the while loop. This loop will execute the code block once, before checking if the condition is true, then it will repeat the loop as long as the condition is true.
Syntax
// code block to be executed
}
while (condition);
Example
The example below uses a do/while
loop. The loop will always be executed at least once, even if the condition is false, because the code block is executed before the condition is tested:
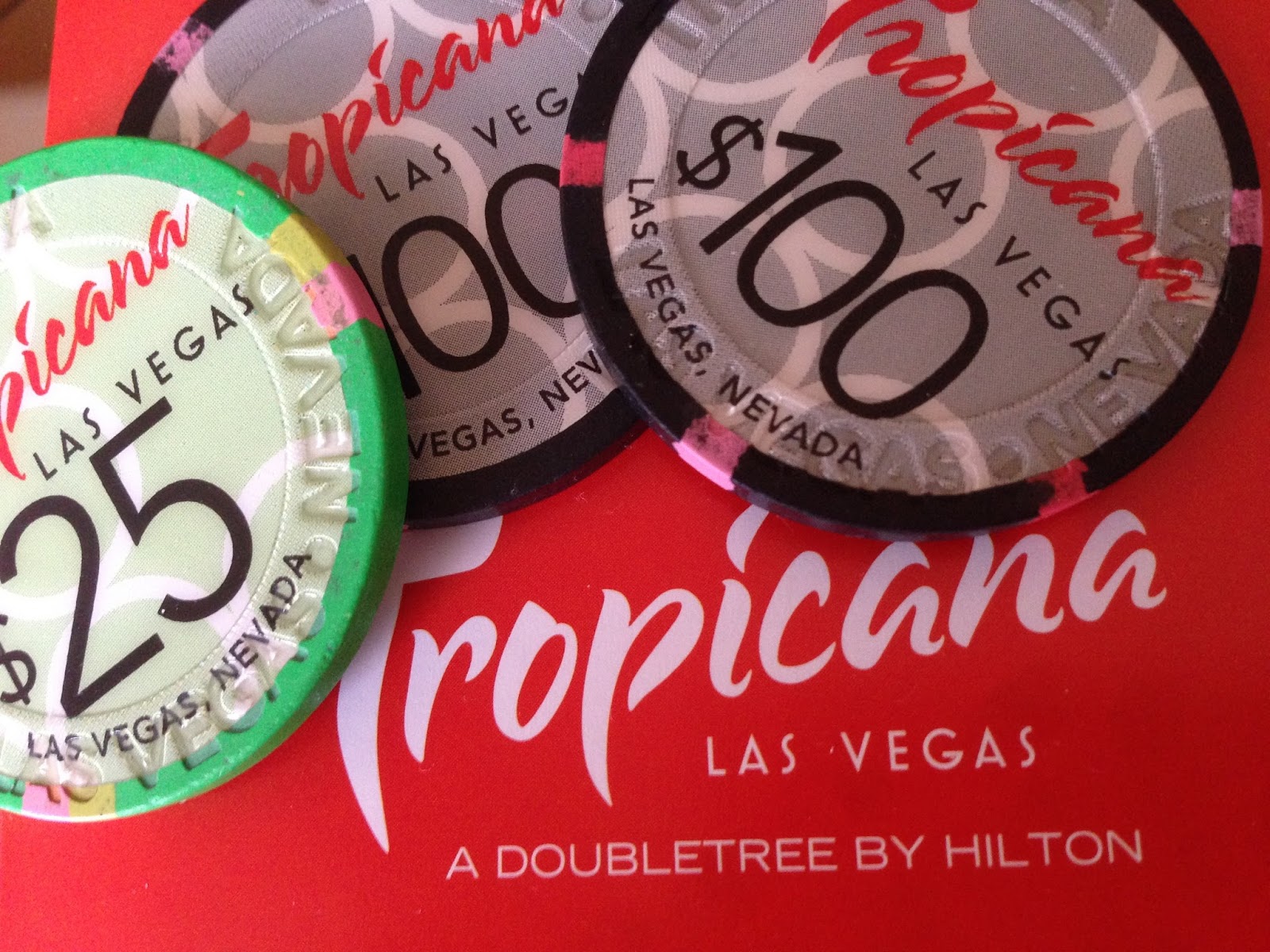
Example
text += 'The number is ' + i;
i++;
}
while (i < 10);
Do not forget to increase the variable used in the condition, otherwise the loop will never end!
Comparing For and While
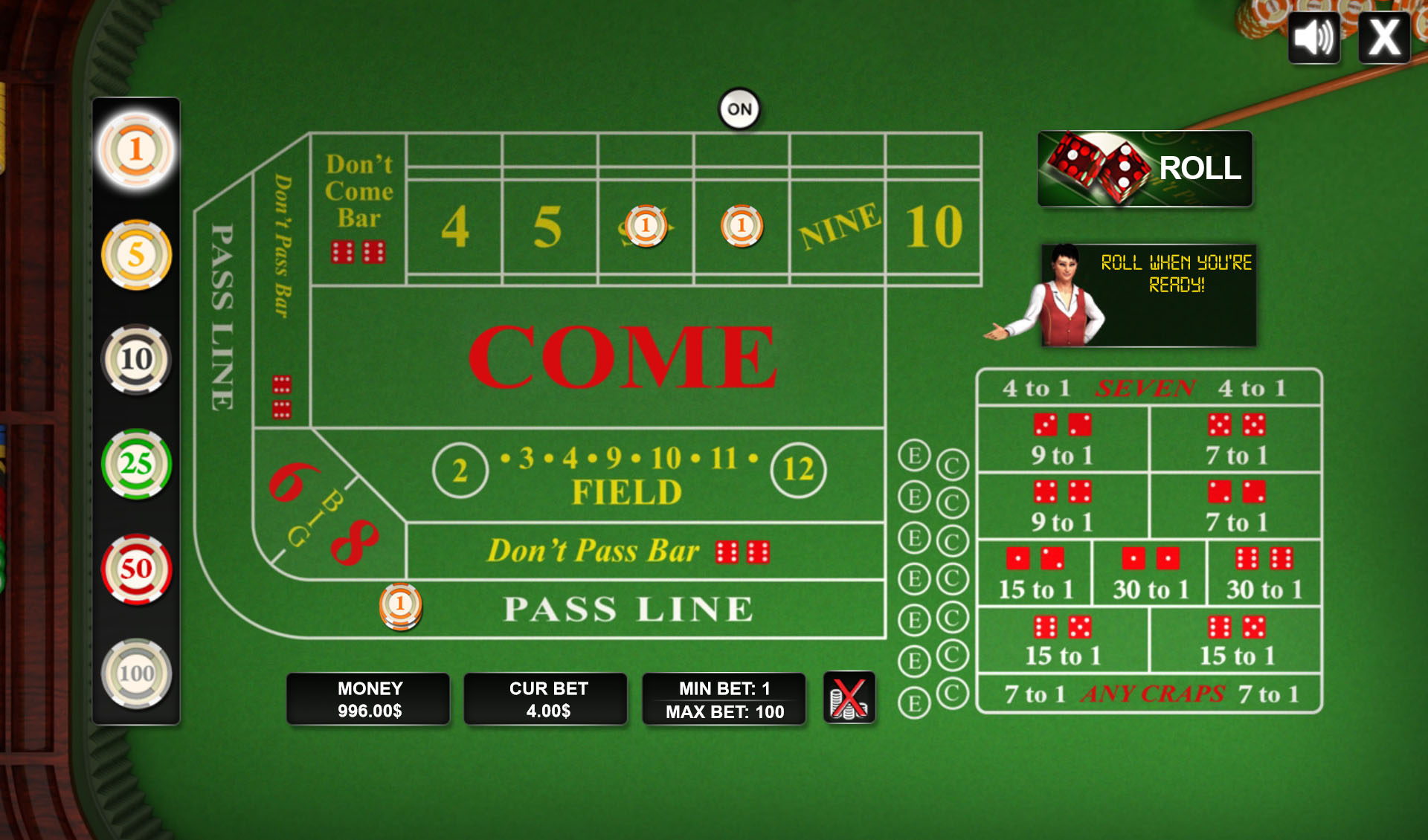
If you have read the previous chapter, about the for loop, you will discover that a while loop is much the same as a for loop, with statement 1 and statement 3 omitted.
The loop in this example uses a for
loop to collect the car names from the cars array:
Example
var i = 0;
var text = ';
for (;cars[i];) {
text += cars[i] + '<br>';
i++;
}
The loop in this example uses a while
loop to collect the car names from the cars array:
Example
Blackjack Javascript Code
var i = 0;
var text = ';
while (cars[i]) {
text += cars[i] + '<br>';
i++;
}
Craps Javascript
Try it Yourself »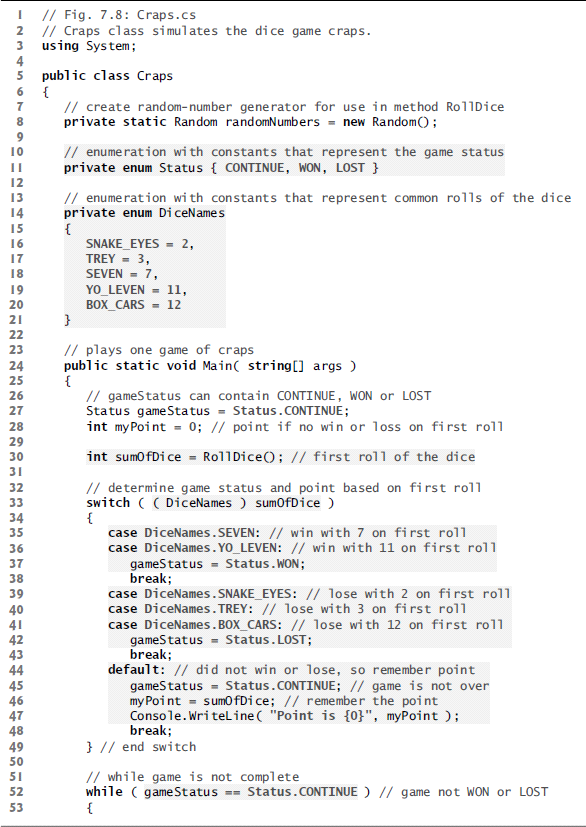